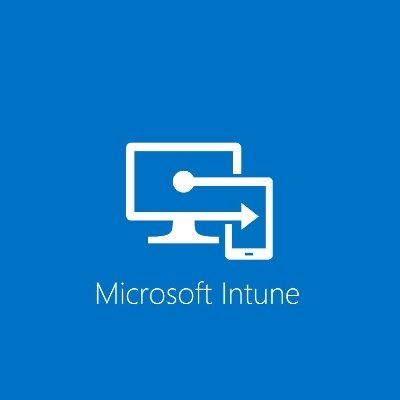
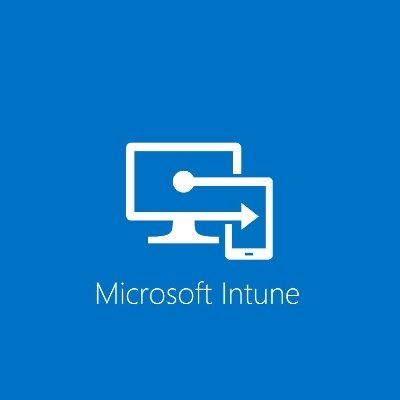
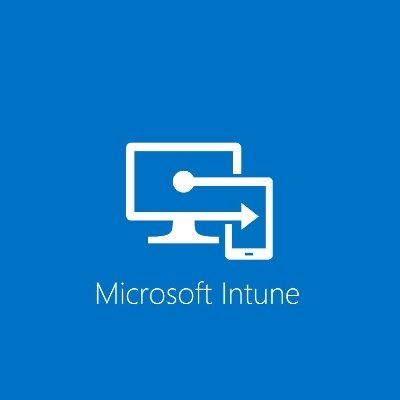
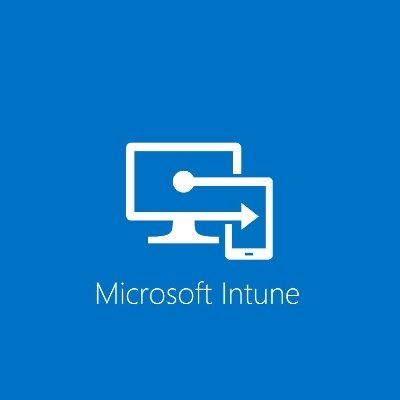
SCCM - MOF Importer V1.0
I had developed a script 4 years ago for a requirement to automate the process
of importing an MOF file to collect custom hardware/software inventory either
with IDMIF and NOIDMIF requirements.
The script is slightly longer than the actual SCCM PowerShell SDK commands to commit
the new classes to SCCM as the other part of the script is able to read an
entire MOF file and calculate how many properties are available and then create
each one within the WMI class space and then commit the changes to SCCM.
For a bullet point review here is what the script can do;
• Read the MOF File
• Calculate all of the properties within the MOF File
• Confirm the WMI namespace
• Parse the MOF File to confirm the properties
• Create the WMI class methods
• Import into SCCM
• Optional lines to create a new Client Settings with the new classes included
Version 2.0
of this script had been
started and will be released soon with other features such as
• Creation of MOF File
• Creation of MIF file for NOIDMIF processes
You can find the script below
#############################################################
#SCCM - MOF Importing Script
# Written By Dujon Walsham
# Updates Included are
# Can now read every property an MOF can have
# Creates properties for total amount of properties detected
# Can handle multiple and singular values for properties
# Site Code now hidden within the variable
##############################################################
#MOF File Location
$MOF = Read-Host "Location of MOF File"
$MOFContent = Get-Content $MOF
$MOFContent = $MOFContent -replace "string\[\]", "Specialstr"
$MOFContent = $MOFContent -replace "uint16\[\]", "Specialui16"
#MOF Parsing
$SMSNamespace = "\\.\\root\\cimv2\\sms"
$SMSNameSpaceCheck = Read-Host "Is this the correct Namespace $SMSNamespace (enter No to change the namespace)"
If ($SMSNamespaceCheck -eq "no") {
$SMSNamespace = Read-Host "Namespace Location i.e. \\.\\root\cimv2\\sms (default is \\.\\root\cimv2)"
}
$SMSClassName = $MOFContent | Where-Object {$_ -like "Class*"}
$SMSGroupName = $MOFContent | Where-Object {$_ -like "*SMS_GROUP_NAME*"}
$SMSClassID = $MOFContent | Where-Object {$_ -like "*SMS_CLASS_ID*"}
$SMSStringProperties = $MOFContent | Where-Object {$_ -like "*String*"}
$SMSBooleanProperties = $MOFContent | Where-Object {$_ -like "*boolean*"}
$SMSUint16Properties = $MOFContent | Where-Object {$_ -like "*Uint16*"}
$SMSUint32Properties = $MOFContent | Where-Object {$_ -like "*Uint32*"}
$SMSDateTimeProperties = $MOFContent | Where-Object {$_ -like "*DateTime*"}
$SMSSpecialstrProperties = $MOFContent | Where-Object {$_ -like "*Specialstr*"}
$SMSSpecialuitProperties = $MOFContent | Where-Object {$_ -like "*specialuit*"}
####################
#Property Parsing
#####################
#Strings
Write-Host The MOF File has detected there are $SMSStringProperties.Count string properties
If ($SMSStringProperties.count -eq "1")
{
ForEach ($line in $SMSStringProperties)
{
For($i=0; $i -le $SMSStringProperties.Count -1; $i++)
{
New-Variable -Name "BooleanPropertyName$i" -Value $SMSStringProperties -Force
}
}
}
Else
{
ForEach ($line in $SMSStringProperties)
{
For($i=0; $i -le $SMSStringProperties.Count -1; $i++)
{
New-Variable -Name "StringPropertyName$i" -Value $SMSStringProperties[$i] -Force
}
}
}
$Strings = For($i=0; $i -le $SMSStringProperties.Count -1; $i++) {Get-Variable -Name StringPropertyName[$i]}
$Strings | ForEach-Object {$_.Name} {Set-Variable -Name $_.Name -Value ($_.Value -replace "string", "" -replace ";", "")}
$Strings | ForEach-Object {$_.Name} {Set-Variable -Name $_.Name -Value ($_.Value.trimstart())}
#Boolean
Write-Host The MOF File has detected there are $SMSBooleanProperties.count boolean properties
If ($SMSBooleanProperties.count -eq "1")
{
ForEach ($line in $SMSBooleanProperties)
{
For($i=0; $i -le $SMSBooleanProperties.Count -1; $i++)
{
New-Variable -Name "BooleanPropertyName$i" -Value $SMSBooleanProperties -Force
}
}
}
Else
{ForEach ($line in $SMSBooleanProperties)
{
For($i=0; $i -le $SMSBooleanProperties.Count -1; $i++)
{
New-Variable -Name "BooleanPropertyName$i" -Value $SMSBooleanProperties[$i] -Force
}
}
}
$Boolean = For($i=0; $i -le $SMSBooleanProperties.Count -1; $i++) {Get-Variable -Name BooleanPropertyName[$i]}
$Boolean | ForEach-Object {$_.Name} {Set-Variable -Name $_.Name -Value ($_.Value -replace "boolean", "" -replace ";", "")}
$Boolean | ForEach-Object {$_.Name} {Set-Variable -Name $_.Name -Value ($_.Value.trimstart())}
#Uint16
Write-Host The MOF File has detected there are $SMSUint16Properties.count Uint16 properties
If ($SMSUint16Properties.count -eq "1")
{
ForEach ($line in $SMSUint16Properties)
{
For($i=0; $i -le $SMSUint16Properties.Count -1; $i++)
{
New-Variable -Name "Uint16PropertyName$i" -Value $SMSUint16Properties -Force
}
}
}
Else
{
ForEach ($line in $SMSUint16Properties)
{
For($i=0; $i -le $SMSUint16Propertiess.Count -1; $i++)
{
New-Variable -Name "Uint16PropertyName$i" -Value $SMSUint16Properties[$i]
}
}
}
$Uint16 = For($i=0; $i -le $SMSUint16Properties.Count -1; $i++) {Get-Variable -Name Uint16PropertyName[$i]}
$Uint16 | ForEach-Object {$_.Name} {Set-Variable -Name $_.Name -Value ($_.Value -replace "Uint16", "" -replace ";", "")}
$Uint16 | ForEach-Object {$_.Name} {Set-Variable -Name $_.Name -Value ($_.Value.trimstart())}
#Uint32
Write-Host The MOF File has detected there are $SMSUint32Properties.count Uint32 properties
If ($SMSUint32Properties.count -eq "1")
{
ForEach ($line in $SMSUint32Properties)
{
For($i=0; $i -le $SMSUint32Properties.Count -1; $i++)
{
New-Variable -Name "Uint32PropertyName$i" -Value $SMSUint32Properties -Force
}
}
}
Else
{
ForEach ($line in $SMSUint32Properties)
{
For($i=0; $i -le $SMSUint32Properties.Count -1; $i++)
{
New-Variable -Name "Uint32PropertyName$i" -Value $SMSUint32Properties[$i]
}
}
}
$Uint32 = For($i=0; $i -le $SMSUint32Properties.Count -1; $i++) {Get-Variable -Name Uint32PropertyName[$i]}
$Uint32 | ForEach-Object {$_.Name} {Set-Variable -Name $_.Name -Value ($_.Value -replace "Uint32", "" -replace ";", "")}
$Uint32 | ForEach-Object {$_.Name} {Set-Variable -Name $_.Name -Value ($_.Value.trimstart())}
#DateTime
Write-Host The MOF File has detected there are $SMSDateTimeProperties.count DateTime properties
If ($SMSDateTimeProperties.count -eq "1")
{
ForEach ($line in $SMSDateTimeProperties)
{
For($i=0; $i -le $SMSDateTimeProperties.Count -1; $i++)
{
New-Variable -Name "DateTimePropertyName$i" -Value $SMSDateTimeProperties -Force
}
}
}
Else
{
ForEach ($line in $SMSDateTimeProperties)
{
For($i=0; $i -le $SMSDateTimeProperties.Count -1; $i++)
{
New-Variable -Name "DateTimePropertyName$i" -Value $SMSDateTimeProperties[$i]
}
}
}
$DateTime = For($i=0; $i -le $SMSDateTimeProperties.Count -1; $i++) {Get-Variable -Name DateTimePropertyName[$i]}
$DateTime | ForEach-Object {$_.Name} {Set-Variable -Name $_.Name -Value ($_.Value -replace "DateTime", "" -replace ";", "")}
$DateTime | ForEach-Object {$_.Name} {Set-Variable -Name $_.Name -Value ($_.Value.trimstart())}
#String[]
Write-Host The MOF File has detected there are $SMSSpecialstrProperties.count Specialstr properties
If ($SMSSpecialstrProperties.count -eq "1")
{
ForEach ($line in $SMSSpecialstrProperties)
{
For($i=0; $i -le $SMSSpecialstrProperties.Count -1; $i++)
{
New-Variable -Name "SpecialstrPropertyName$i" -Value $SMSSpecialstrProperties -Force
}
}
}
Else
{
ForEach ($line in $SMSSpecialstrProperties)
{
For($i=0; $i -le $SMSSpecialstrProperties.Count -1; $i++)
{
New-Variable -Name "SpecialstrPropertyName$i" -Value $SMSSpecialstrProperties[$i]
}
}
}
$Specialstr = For($i=0; $i -le $SMSSpecialstrProperties.Count -1; $i++) {Get-Variable -Name SpecialstrPropertyName[$i]}
$Specialstr | ForEach-Object {$_.Name} {Set-Variable -Name $_.Name -Value ($_.Value -replace "Specialstr", "" -replace ";", "")}
$Specialstr | ForEach-Object {$_.Name} {Set-Variable -Name $_.Name -Value ($_.Value.trimstart())}
#Uint16[]
Write-Host The MOF File has detected there are $SMSSpecialuitProperties.count Specialuit properties
If ($SMSSpecialuitProperties.count -eq "1")
{
ForEach ($line in $SMSSpecialuitProperties)
{
For($i=0; $i -le $SMSSpecialuitProperties.Count -1; $i++)
{
New-Variable -Name "SpecialuitPropertyName$i" -Value $SMSSpecialuitProperties -Force
}
}
}
Else
{
ForEach ($line in $SMSSpecialuitProperties)
{
For($i=0; $i -le $SMSSpecialuitProperties.Count -1; $i++)
{
New-Variable -Name "SpecialuitPropertyName$i" -Value $SMSSpecialuitProperties[$i]
}
}
}
$Specialuit = For($i=0; $i -le $SMSSpecialuitProperties.Count -1; $i++) {Get-Variable -Name SpecialuitPropertyName[$i]}
$Specialuit | ForEach-Object {$_.Name} {Set-Variable -Name $_.Name -Value ($_.Value -replace "Specialuit", "" -replace ";", "")}
$Specialuit | ForEach-Object {$_.Name} {Set-Variable -Name $_.Name -Value ($_.Value.trimstart())}
#Further MOF Parsing
$SMSClassName = $SMSClassName -replace "\:", "" -replace "SMS_Class_Template", "" -replace "Class", ""
$SMSClassName = $SMSClassName.TrimStart()
$SMSGROUPNAME = $SMSGROUPNAME.TrimStart() -replace "SMS_GROUP_NAME" , "" -replace ",", "" -replace "\(", "" -replace "\)", ""
$SMSGROUPNAME = $SMSGROUPNAME.TrimStart()
$SMSGroupName = $SMSGROUPNAME.Replace("`"", "")
$SMSClassID = $SMSClassID.TrimStart() -replace "SMS_CLASS_ID", "" -replace "\]", "" -replace "\(" -replace "\)"
$SMSClassID = $SMSClassID.TrimStart()
$SMSClassID = $SMSClassID.Replace("`"", "")
#Confirm MOF Settings
Write-Host Please check the following details are correct
Write-Host The Namespace is $SMSNamespace
Write-Host The Class Name is $SMSClassName
Write-Host The SMS Group Name is $SMSGroupName
Write-Host The SMS Class ID is $SMSClassID
$Strings | ForEach-Object {$_.Value} {Write-Host The MOF file has $SMSStringProperties.Count string properties which are $_.Value}
$Boolean | ForEach-Object {$_.Value} {Write-Host The MOF file has $SMSBooleanProperties.Count boolean properties which are $_.Value}
$Uint16 | ForEach-Object {$_.Value} {Write-Host The MOF file has $SMSUint16Properties.Count Uint16 properties which are $_.Value}
$Uint32 | ForEach-Object {$_.Value} {Write-Host The MOF file has $SMSUint32Properties.Count Uint32 properties which are $_.Value}
$DateTime | ForEach-Object {$_.Value} {Write-Host The MOF file has $SMSDateTimeProperties.Count DateTime properties which are $_.Value}
$Specialstr | ForEach-Object {$_.Value} {Write-Host The MOF file has $SMSSpecialstrProperties.Count Specialstr properties which are $_.Value}
$Specialuit | ForEach-Object {$_.Value} {Write-Host The MOF file has $SMSSpecialuitProperties.Count Specialuit properties which are $_.Value}
Read-Host Press any key to proceed in building the MOF in WMI
###################################
#Convert MOF File to MOF WMI
####################################
#Build Inventory Class WMI Table
$SiteCode = Get-WmiObject -ComputerName $Env:computername -Namespace "Root\sms" -Property SiteCode -Class "SMS_ProviderLocation" | ForEach-Object {$_.SiteCode}
$invclassPropClass = "[wmiclass]""root\sms\site_$sitecode" + ":SMS_InventoryClassProperty" + """"
$invclassPropClass = Invoke-Expression $InvClassPropClass
#Count Number of Properties needed to create
$Total = $SMSStringProperties.count + $SMSBooleanProperties.count + $SMSUint16Properties.count + $SMSUint32Properties.count + $SMSDateTimeProperties.count + $SMSSpecialstrProperties.count + $SMSSpecialuitProperties.count
Write-Host The current MOF has $Total properties in total
#Strings Properties
For($i=0; $i -le $SMSStringProperties.Count -1; $i++) {New-Variable -Name "StringProperty$i" -Value $InvClassPropClass.CreateInstance()}
$StringPropertiesName = For($i=0; $i -le $SMSStringProperties.Count -1; $i++) {Get-Variable -Name "StringProperty$i"}
$StringPropertiesName = $StringPropertiesName | ForEach-Object {"$" + $_.Name + ".PropertyName" + " =" + " " + "$" + "R" + $_.Name -replace "RStringProperty", "StringPropertyName" }
$StringPropertiesName = $StringPropertiesName | Out-String
Invoke-Expression $StringPropertiesName
$StringPropertiesIsKey = For($i=0; $i -le $SMSStringProperties.Count -1; $i++) {Get-Variable -Name "StringProperty$i"}
$StringPropertiesIsKey = $StringPropertiesIsKey | ForEach-Object {"$" + $_.Name + ".IsKey" + " =" + " " + "$" + $False}
$StringPropertiesIsKey = $StringPropertiesIsKey | Out-String
Invoke-Expression $StringPropertiesIsKey
$StringPropertiesType = For($i=0; $i -le $SMSStringProperties.Count -1; $i++) {Get-Variable -Name "StringProperty$i"}
$StringPropertiesType = $StringPropertiesType | ForEach-Object {"if (" + "$" + "Strings.value -contains" + " $" + $_.Name + ".PropertyName)" + " {" + "$" + $_.Name + ".Type = 8}" }
$StringPropertiesType = $StringPropertiesType | Out-String
Invoke-Expression $StringPropertiesType
#Boolean Properties
For($i=0; $i -le $SMSBooleanProperties.Count -1; $i++) {New-Variable -Name "BooleanProperty$i" -Value $InvClassPropClass.CreateInstance()}
$BooleanPropertiesName = For($i=0; $i -le $SMSBooleanProperties.Count -1; $i++) {Get-Variable -Name "BooleanProperty$i"}
$BooleanPropertiesName = $BooleanPropertiesName | ForEach-Object {"$" + $_.Name + ".PropertyName" + " =" + " " + "$" + "R" + $_.Name -replace "RBooleanProperty", "BooleanPropertyName" }
$BooleanPropertiesName = $BooleanPropertiesName | Out-String
Invoke-Expression $BooleanPropertiesName
$BooleanPropertiesIsKey = For($i=0; $i -le $SMSBooleanProperties.Count -1; $i++) {Get-Variable -Name "BooleanProperty$i"}
$BooleanPropertiesIsKey = $BooleanPropertiesIsKey | ForEach-Object {"$" + $_.Name + ".IsKey" + " =" + " " + "$" + $False}
$BooleanPropertiesIsKey = $BooleanPropertiesIsKey | Out-String
Invoke-Expression $BooleanPropertiesIsKey
$BooleanPropertiesType = For($i=0; $i -le $SMSBooleanProperties.Count -1; $i++) {Get-Variable -Name "BooleanProperty$i"}
$BooleanPropertiesType = $BooleanPropertiesType | ForEach-Object {"if (" + "$" + "Boolean.value -contains" + " $" + $_.Name + ".PropertyName)" + " {" + "$" + $_.Name + ".Type = 11}" }
$BooleanPropertiesType = $BooleanPropertiesType | Out-String
Invoke-Expression $BooleanPropertiesType
#Uint16 Properties
For($i=0; $i -le $SMSUint16Properties.Count -1; $i++) {New-Variable -Name "Uint16Property$i" -Value $InvClassPropClass.CreateInstance()}
$Uint16PropertiesName = For($i=0; $i -le $SMSUint16Properties.Count -1; $i++) {Get-Variable -Name "Uint16Property$i"}
$Uint16PropertiesName = $Uint16PropertiesName | ForEach-Object {"$" + $_.Name + ".PropertyName" + " =" + " " + "$" + "R" + $_.Name -replace "RUint16Property", "Uint16PropertyName" }
$Uint16PropertiesName = $Uint16PropertiesName | Out-String
Invoke-Expression $Uint16PropertiesName
$Uint16PropertiesIsKey= For($i=0; $i -le $SMSUint16Properties.Count -1; $i++) {Get-Variable -Name "Uint16Property$i"}
$Uint16PropertiesIsKey = $Uint16PropertiesIsKey | ForEach-Object {"$" + $_.Name + ".IsKey" + " =" + " " + "$" + $False}
$Uint16PropertiesIsKey = $Uint16PropertiesIsKey | Out-String
Invoke-Expression $Uint16PropertiesIsKey
$Uint16PropertiesType = For($i=0; $i -le $SMSUint16Properties.Count -1; $i++) {Get-Variable -Name "Uint16Property$i"}
$Uint16PropertiesType = $Uint16PropertiesType | ForEach-Object {"if (" + "$" + "Uint16.value -contains" + " $" + $_.Name + ".PropertyName)" + " {" + "$" + $_.Name + ".Type = 18}" }
$Uint16PropertiesType = $Uint16PropertiesType | Out-String
Invoke-Expression $Uint16PropertiesType
#Uint32 Properties
For($i=0; $i -le $SMSUint32Properties.Count -1; $i++) {New-Variable -Name "Uint32Property$i" -Value $InvClassPropClass.CreateInstance()}
$Uint32PropertiesName = For($i=0; $i -le $SMSUint32Properties.Count -1; $i++) {Get-Variable -Name "Uint32Property$i"}
$Uint32PropertiesName = $Uint32PropertiesName | ForEach-Object {"$" + $_.Name + ".PropertyName" + " =" + " " + "$" + "R" + $_.Name -replace "RUint32Property", "Uint32PropertyName" }
$Uint32PropertiesName = $Uint32PropertiesName | Out-String
Invoke-Expression $Uint32PropertiesName
$Uint32PropertiesIsKey= For($i=0; $i -le $SMSUint32Properties.Count -1; $i++) {Get-Variable -Name "Uint32Property$i"}
$Uint32PropertiesIsKey = $Uint32PropertiesIsKey | ForEach-Object {"$" + $_.Name + ".IsKey" + " =" + " " + "$" + $False}
$Uint32PropertiesIsKey = $Uint32PropertiesIsKey | Out-String
Invoke-Expression $Uint32PropertiesIsKey
$Uint32PropertiesType = For($i=0; $i -le $SMSUint32Properties.Count -1; $i++) {Get-Variable -Name "Uint32Property$i"}
$Uint32PropertiesType = $Uint32PropertiesType | ForEach-Object {"if (" + "$" + "Uint32.value -contains" + " $" + $_.Name + ".PropertyName)" + " {" + "$" + $_.Name + ".Type = 19}" }
$Uint32PropertiesType = $Uint32PropertiesType | Out-String
Invoke-Expression $Uint32PropertiesType
#DateTime Properties
For($i=0; $i -le $SMSDateTimeProperties.Count -1; $i++) {New-Variable -Name "DateTimeProperty$i" -Value $InvClassPropClass.CreateInstance()}
$DateTimePropertiesName = For($i=0; $i -le $SMSDateTimeProperties.Count -1; $i++) {Get-Variable -Name "DateTimeProperty$i"}
$DateTimePropertiesName = $DateTimePropertiesName | ForEach-Object {"$" + $_.Name + ".PropertyName" + " =" + " " + "$" + "R" + $_.Name -replace "RDateTimeProperty", "DateTimePropertyName" }
$DateTimePropertiesName = $DateTimePropertiesName | Out-String
Invoke-Expression $DateTimePropertiesName
$DateTimePropertiesIsKey= For($i=0; $i -le $SMSDateTimeProperties.Count -1; $i++) {Get-Variable -Name "DateTimeProperty$i"}
$DateTimePropertiesIsKey = $DateTimePropertiesIsKey | ForEach-Object {"$" + $_.Name + ".IsKey" + " =" + " " + "$" + $False}
$DateTimePropertiesIsKey = $DateTimePropertiesIsKey | Out-String
Invoke-Expression $DateTimePropertiesIsKey
$DateTimePropertiesType = For($i=0; $i -le $SMSDateTimeProperties.Count -1; $i++) {Get-Variable -Name "DateTimeProperty$i"}
$DateTimePropertiesType = $DateTimePropertiesType | ForEach-Object {"if (" + "$" + "DateTime.value -contains" + " $" + $_.Name + ".PropertyName)" + " {" + "$" + $_.Name + ".Type = 101}" }
$DateTimePropertiesType = $DateTimePropertiesType | Out-String
Invoke-Expression $DateTimePropertiesType
#String[] String 1 Properties
For($i=0; $i -le $SMSSpecialstrProperties.Count -1; $i++) {New-Variable -Name "SpecialstrProperty$i" -Value $InvClassPropClass.CreateInstance()}
$SpecialstrPropertiesName = For($i=0; $i -le $SMSSpecialstrProperties.Count -1; $i++) {Get-Variable -Name "SpecialstrProperty$i"}
$SpecialstrPropertiesName = $SpecialstrPropertiesName | ForEach-Object {"$" + $_.Name + ".PropertyName" + " =" + " " + "$" + "R" + $_.Name -replace "RSpecialstrProperty", "SpecialstrPropertyName" }
$SpecialstrPropertiesName = $SpecialstrPropertiesName | Out-String
Invoke-Expression $SpecialstrPropertiesName
$SpecialstrPropertiesIsKey= For($i=0; $i -le $SMSSpecialstrProperties.Count -1; $i++) {Get-Variable -Name "SpecialstrProperty$i"}
$SpecialstrPropertiesIsKey = $SpecialstrPropertiesIsKey | ForEach-Object {"$" + $_.Name + ".IsKey" + " =" + " " + "$" + $False}
$SpecialstrPropertiesIsKey = $SpecialstrPropertiesIsKey | Out-String
Invoke-Expression $SpecialstrPropertiesIsKey
$SpecialstrPropertiesType = For($i=0; $i -le $SMSSpecialstrProperties.Count -1; $i++) {Get-Variable -Name "SpecialstrProperty$i"}
$SpecialstrPropertiesType = $SpecialstrPropertiesType | ForEach-Object {"if (" + "$" + "Specialstr.value -contains" + " $" + $_.Name + ".PropertyName)" + " {" + "$" + $_.Name + ".Type = 8200}" }
$SpecialstrPropertiesType = $SpecialstrPropertiesType | Out-String
Invoke-Expression $SpecialstrPropertiesType
#Uint16[] Specialuit Properties
For($i=0; $i -le $SMSSpecialuitProperties.Count -1; $i++) {New-Variable -Name "SpecialuitProperty$i" -Value $InvClassPropClass.CreateInstance()}
$SpecialuitPropertiesName = For($i=0; $i -le $SMSSpecialuitProperties.Count -1; $i++) {Get-Variable -Name "SpecialuitProperty$i"}
$SpecialuitPropertiesName = $SpecialuitPropertiesName | ForEach-Object {"$" + $_.Name + ".PropertyName" + " =" + " " + "$" + "R" + $_.Name -replace "RSpecialuitProperty", "SpecialuitPropertyName" }
$SpecialuitPropertiesName = $SpecialuitPropertiesName | Out-String
Invoke-Expression $SpecialuitPropertiesName
$SpecialuitPropertiesIsKey= For($i=0; $i -le $SMSSpecialuitProperties.Count -1; $i++) {Get-Variable -Name "SpecialuitProperty$i"}
$SpecialuitPropertiesIsKey = $SpecialuitPropertiesIsKey | ForEach-Object {"$" + $_.Name + ".IsKey" + " =" + " " + "$" + $False}
$SpecialuitPropertiesIsKey = $SpecialuitPropertiesIsKey | Out-String
Invoke-Expression $SpecialuitPropertiesIsKey
$SpecialuitPropertiesType = For($i=0; $i -le $SMSSpecialuitProperties.Count -1; $i++) {Get-Variable -Name "SpecialuitProperty$i"}
$SpecialuitPropertiesType = $SpecialuitPropertiesType | ForEach-Object {"if (" + "$" + "Specialuit.value -contains" + " $" + $_.Name + ".PropertyName)" + " {" + "$" + $_.Name + ".Type = 8210}" }
$SpecialuitPropertiesType = $SpecialuitPropertiesType | Out-String
Invoke-Expression $SpecialuitPropertiesType
#Define the WMI Class
#$InventoryClass = [wmiclass]"root\sms\site_ca1:SMS_InventoryClass"
$InventoryClass = "[wmiclass]""root\sms\site_$sitecode" + ":SMS_InventoryClass" + """"
$InventoryClass = Invoke-Expression $InventoryClass
$NewInvClass = $InventoryClass.CreateInstance()
$NewInvClass.IsDeletable = $true
$NewInvClass.ClassName = $SMSClassName
$NewInvClass.Namespace = $SMSNamespace
$NewInvClass.SMSClassID = $SMSClassID
$NewInvClass.SMSGroupName = $SMSGroupName
#Add Properties to Class Definition
$StringClassDefinition = For($i=0; $i -le $SMSStringProperties.Count -1; $i++) {Get-Variable -Name "StringProperty$i"}
$StringClassDefinition = $StringClassDefinition | ForEach-Object {"$" + "NewInvClass.Properties += [System.Management.ManagementObject]" + "$" + $_.Name }
$StringClassDefinition = $StringClassDefinition | Out-String
Invoke-Expression $StringClassDefinition
$BooleanClassDefinition = For($i=0; $i -le $SMSBooleanProperties.Count -1; $i++) {Get-Variable -Name "BooleanProperty$i"}
$BooleanClassDefinition = $BooleanClassDefinition | ForEach-Object {"$" + "NewInvClass.Properties += [System.Management.ManagementObject]" + "$" + $_.Name }
$BooleanClassDefinition = $BooleanClassDefinition | Out-String
Invoke-Expression $BooleanClassDefinition
$Uint16ClassDefinition = For($i=0; $i -le $SMSUint16Properties.Count -1; $i++) {Get-Variable -Name "Uint16Property$i"}
$Uint16ClassDefinition = $Uint16ClassDefinition | ForEach-Object {"$" + "NewInvClass.Properties += [System.Management.ManagementObject]" + "$" + $_.Name }
$Uint16ClassDefinition = $Uint16ClassDefinition | Out-String
Invoke-Expression $Uint16ClassDefinition
$Uint32ClassDefinition = For($i=0; $i -le $SMSUint32Properties.Count -1; $i++) {Get-Variable -Name "Uint32Property$i"}
$Uint32ClassDefinition = $Uint32ClassDefinition | ForEach-Object {"$" + "NewInvClass.Properties += [System.Management.ManagementObject]" + "$" + $_.Name }
$Uint32ClassDefinition = $Uint32ClassDefinition | Out-String
Invoke-Expression $Uint32ClassDefinition
$DateTimeClassDefinition = For($i=0; $i -le $SMSDateTimeProperties.Count -1; $i++) {Get-Variable -Name "DateTimeProperty$i"}
$DateTimeClassDefinition = $DateTimeClassDefinition | ForEach-Object {"$" + "NewInvClass.Properties += [System.Management.ManagementObject]" + "$" + $_.Name }
$DateTimeClassDefinition = $DateTimeClassDefinition | Out-String
Invoke-Expression $DateTimeClassDefinition
$SpecialstrClassDefinition = For($i=0; $i -le $SMSSpecialstrProperties.Count -1; $i++) {Get-Variable -Name "SpecialstrProperty$i"}
$SpecialstrClassDefinition = $SpecialstrClassDefinition | ForEach-Object {"$" + "NewInvClass.Properties += [System.Management.ManagementObject]" + "$" + $_.Name }
$SpecialstrClassDefinition = $SpecialstrClassDefinition | Out-String
Invoke-Expression $SpecialstrClassDefinition
$SpecialuitClassDefinition = For($i=0; $i -le $SMSSpecialuitProperties.Count -1; $i++) {Get-Variable -Name "SpecialuitProperty$i"}
$SpecialuitClassDefinition = $SpecialuitClassDefinition | ForEach-Object {"$" + "NewInvClass.Properties += [System.Management.ManagementObject]" + "$" + $_.Name }
$SpecialuitClassDefinition = $SpecialuitClassDefinition | Out-String
Invoke-Expression $SpecialuitClassDefinition
# Commit the inventory class instance to the provider
$NewInvClass.Put()
#Create Custom Client Settings
Import-Module -Name "$(split-path $Env:SMS_ADMIN_UI_PATH)\ConfigurationManager.psd1"
Set-Location $SiteCode
New-CMClientSetting -Name $SiteCode Hardware Inventory Extensions -Type Device
Set-CMClientSetting -HardwareInventorySettings -EnableHardwareInventory $True -Name $SiteCode Hardware Inventory Extensions
Write-Host Please enable the $SMSGroupName within the new Custom Client Settings. Once done press any key to continue
#Deploy Custom Client Settings
$CollectionName = Read-Host "Name of Collection to deploy to"
Start-CMClientSettingDeployment -ClientSettingName $SiteCode Hardware Inventory Extensions -CollectionName $CollectionName